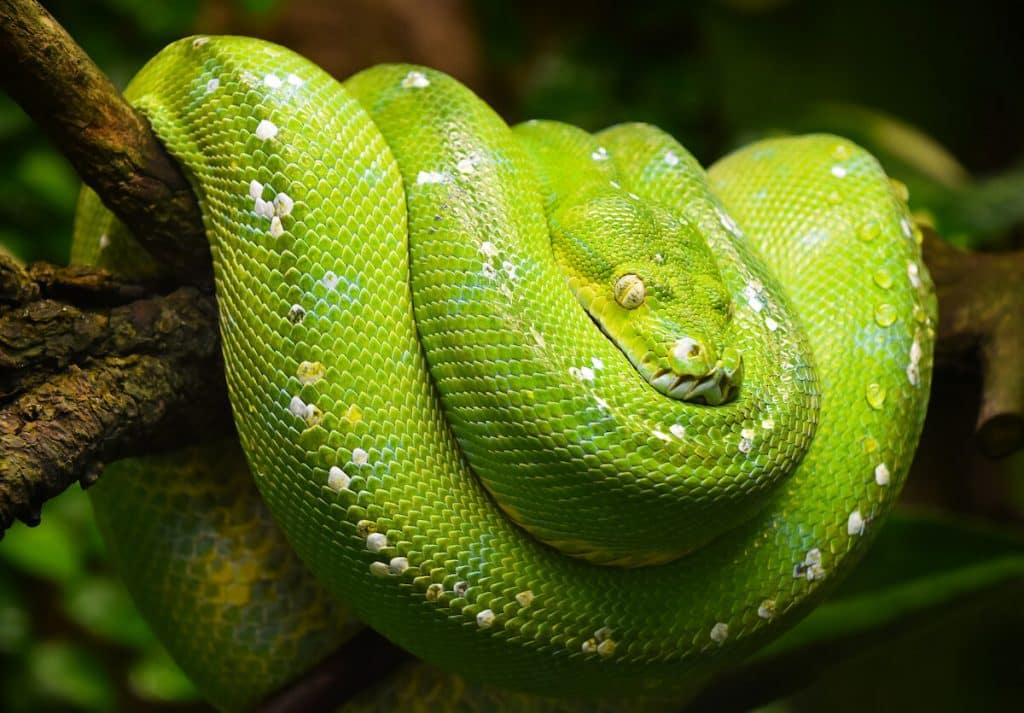
There are a lot of tools you need to successfully operate in markets, from solid mathematical skills to the ability to do extensive research into company fundamentals.
Over the past few decades, programming has become increasingly important. Though not all traders are programmers, being able to write good code is the kind of thing that will set you apart and make you a valued member of a trading firm.
There are thousands of different programming languages, but among the most popular is the Python programming language. Today, we’re going to answer some basic questions about Python in finance, such as how it’s used and why it’s become so popular. Armed with this information, you’ll be able to decide whether you want to devote the effort required to add “programmer” to your resume.
What Is Python?
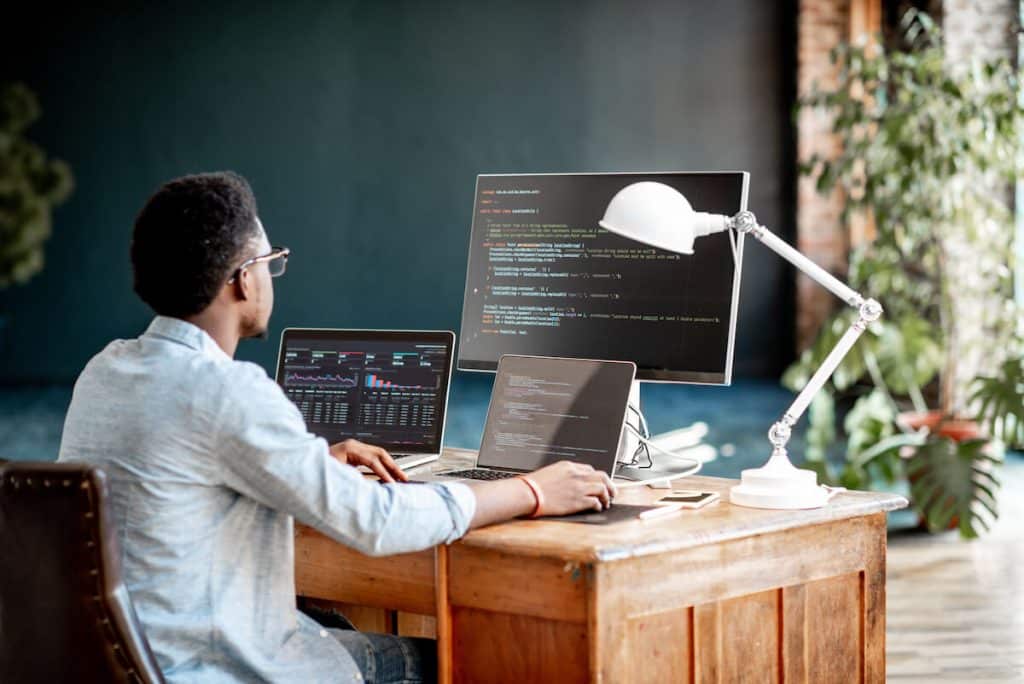
Python is an open-source programming language with a clean, readable syntax, and a gentle learning curve that makes it easy to pick up the fundamentals from basic tutorials. Add to this a near-infinite number of Python libraries (also known as “Python packages” or “modules”) aimed at different use cases, and it’s not hard to see why data scientists, machine learning and deep learning engineers, and quants doing everything from algorithmic trading to derivatives analysis opt to use Python.
To validate our claim that Python code is extremely easy to read, let’s take a basic example:
for name in list_of_names:
print(name)
Even if you’ve never seen a line of code before, you can probably immediately tell exactly how this Python code works and what it’s meant to accomplish. Now, admittedly this is due in part to the simplicity of the example and the fact that we’ve chosen good variable names. Still, Python is famously readable, and equivalent code in Java or Haskell would be much more intimidating.
It’s also worth mentioning that Python is what is known as an “object-oriented programming language” (OOP). This is a term you will have encountered if you’ve studied any computer science, and it’s definitely something you’ll be reading about if you do any tutorials on the Python programming language.
In a nutshell, “objects” are the basic organizational unit of the language. This distinguishes it from other approaches to programming language design that put, say, functions center stage.
In Python, everything is an object. This means that even the simplest data structures (like a variable storing my name) will have both associated metadata and a set of methods that can be used to manipulate them.
Don’t worry, it’s easy to see what we mean with an example. Let’s put my name in a variable like we discussed:
my_name = “Rishi Singh”
(If it helps, you can think of the “my_name” variable as being like a box with a bunch of buttons and knobs that allow you to do useful things to whatever information it contains.)
What might these “useful things” consist of? Well, suppose that we want to lowercase my name, what would you call such a method? How about “lower”? In the Python programming language, this is exactly how we lowercase a string:
my_name.lower()
What if we’re curious about how many “i”s my name contains? What if, in other words, we want to count the number of times the letter “i“ shows up in “Rishi Singh”? You guessed it, we can get this done with an aptly-named method:
my_name.count(“i”)
It’s hard to beat this kind of simplicity when thinking about a programming language to learn. Now that we’ve covered this ground, let’s turn to some major use cases for Python.
Data Science With Python
It wouldn’t be much of an exaggeration to say that Python is the programming language for data science. There are teams that work with Java, Rust, and (sometimes) Scala, but if you chose a data science team at random, there’s at least a 90% chance that they’re using Python.
Part of this is because Python has native support for so many different data types. Out of the box, it comes with tons of methods for working data structures like arrays.
But there are also Python-based modules like Pandas that make financial data analysis much easier than it would be with a tool like Microsoft’s Excel. Pandas works on dataframes — which are redolent of spreadsheets — and offer all sorts of easy methods for handling data manipulation tasks. If you want to do data processing work, like changing the data types in your columns, using anonymous lambda functions for more involved operations, making pivot tables, or anything else, it’ll be pretty easy in Pandas.
There’s even a way now to pass in SQL queries if you’re more comfortable with that.
One drawback to Pandas is that it can be pretty slow. If latency is a huge concern, you might have more luck with the leaner numpy package, which is also better suited to scientific computing applications or anything else that relies on lightning-fast operations performed over vectors.
Data Visualization With Python
Data visualization is another use case for Python in finance. For basic data visualization tasks, you’re probably going to be using the standard tool, matplotlib. For more customization or for creating interactive plots, Plotly or Altair will probably serve you better.
Machine Learning With Python
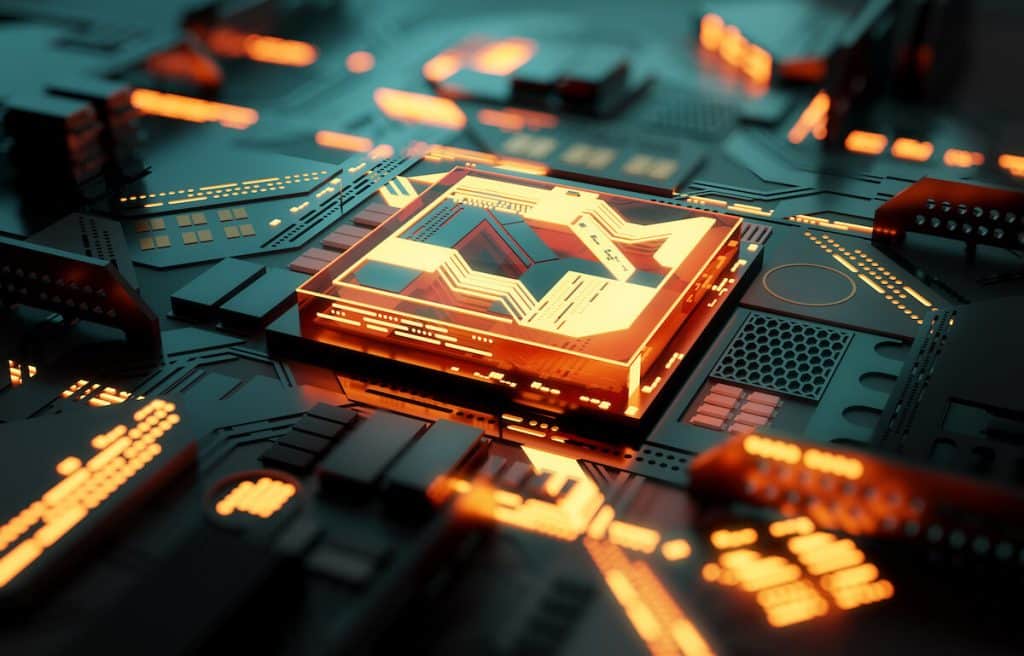
To simplify a great deal, machine learning refers to the set of techniques by which machines can derive statistical patterns from data. You could build a simple machine learning model to predict future returns on stock time series data, for example, and then create a trading strategy around it.
The main library for machine learning in Python is scikit-learn (typically abbreviated as “sklearn”), which offers a simple interface for building linear and logistic regressions, random forests, and ensemble models, among other things.
Deep Learning With Python
If you find that the suite of basic machine-learning models simply isn’t powerful enough to accomplish what you want, it’s time to consider building a deep-learning model. “Deep learning” almost always means “neural networks,” and the Tensorflow library is among the most popular ways of building out and training a neural network with Python.
How Does Python in Finance Work?
Now that we’ve covered these essentials, let’s turn our attention to some specifics. When it comes to Python, finance is an ideal use case. And, in fact, many financial institutions are looking for better ways to leverage their financial data for modeling, algorithmic trading, and tons of other use cases, and it’s worth understanding what this actually looks like.
Financial Analysis With Python
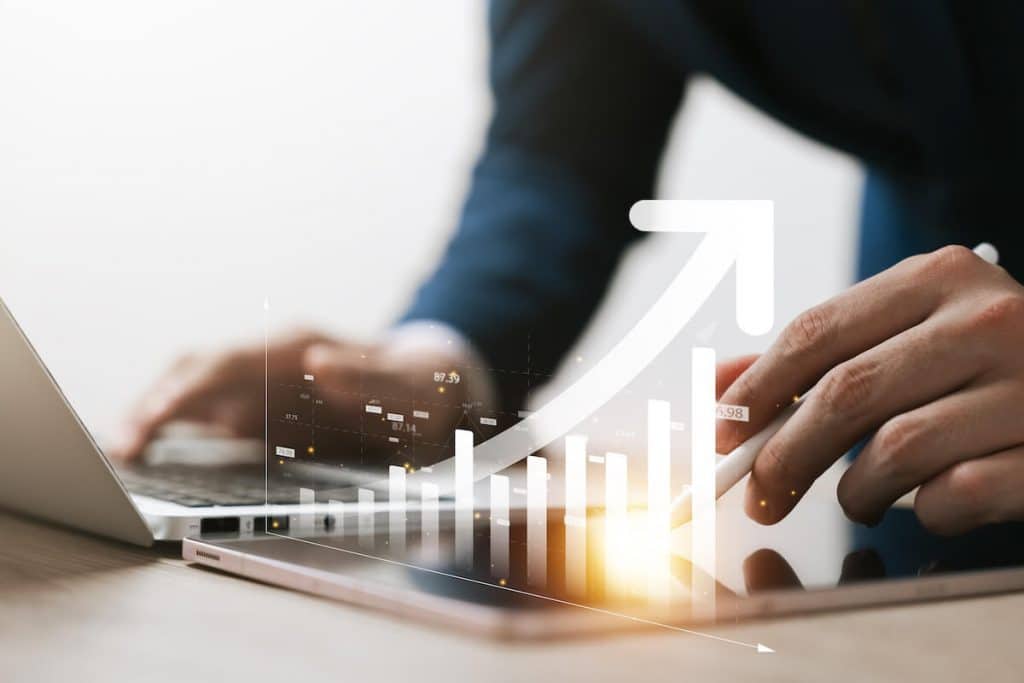
Perhaps the simplest way to leverage Python programming for finance is with financial analysis. You might pull asset pricing datasets down through an API to look for correlations, for example, or to visualize the past few month’s worth of returns.
You can do all of this in a proper Python script, but if you’re just dipping your toes in the water, you might consider using a Jupyter notebook for your financial analytics instead. This makes it much easier to get started without having to worry about setting up an integrated development environment (IDE).
Financial Modeling With Python
When you get into doing more advanced quantitative finance, you’ll also find Python to be a suitable tool. We’ll discuss a few ways this works in this section, but first let’s dwell for a moment on the fact that financial data almost always consists of time series data, so there are special considerations.
Time series data is more or less what it sounds like — it’s data points arrayed across time. This means you have to be careful about the way you, for example, feed it into a machine learning model. In some circumstances, it’s standard practice to shuffle data around before it’s used to train a machine learning model, but you can’t do that with time series data because future information will get mixed in with past data, thus undermining the ability of your model to generalize.
A basic financial modeling task you can do with Python is calculating the volatility of returns data for a particular stock. As it happens, numpy has a built-in method “std” with which you can do this.
Algorithmic Trading With Python
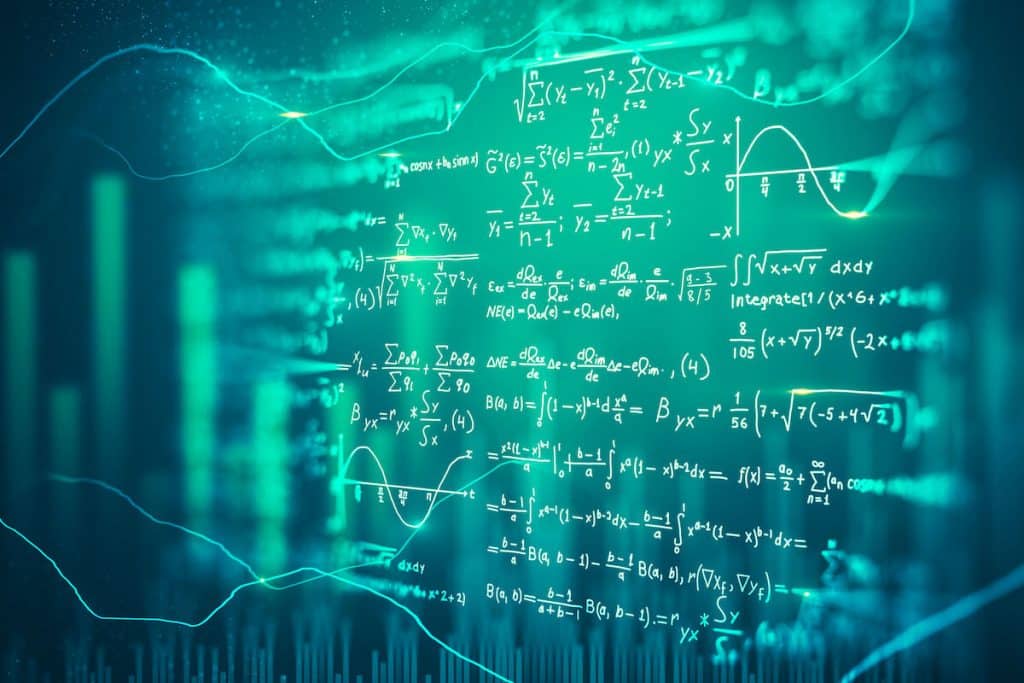
“Algorithmic trading” refers to the creation of mostly or fully automated trading strategies that rely heavily on statistical models, artificial intelligence, or machine learning to execute lightning-fast trades when certain conditions are met. Algorithmic trading was actually born of the realization that tremendous amounts of money could be made with the construction of algorithms that could respond in real time to changing market structure far more quickly than a human could ever hope to.
Today, algorithmic trading remains highly contentious. There are those who believe it forms an integral part of the capital markets function and is mostly benign, and those that worry not only about the possibility of increasingly complex chains of decisions of which humans are not a part, but of the possibility that these algorithms could contribute to instability.
Wherever you come down on this, it’s worth understanding algorithmic trading and how it can be accomplished with the Python programming language. Because Python is so important to the financial industry in general, it probably won’t shock you to learn that there are many resources for those who want to build algorithmic trading systems with it.
There are plenty of high-quality tutorials, of course, but you can also dive into one of the many book-length treatments of the subject as well. Yves Hilpisch’s “Python for Algorithmic Trading“ from O’Reilly comes highly recommended and should be an excellent resource.
Just be careful. Trading is hard, and algorithmic trading is one of the hardest approaches. Remember that if it’s possible to make $70,000 in five minutes with a bot, it’s also possible to lose that much just as quickly.
Wrapping Your Head Around Python for Finance
Programming in languages like Python is an important part of finance and will likely only become more so in the future. From data modeling to algorithmic trading and beyond, learning how to read and write the language of code will give you an edge as you navigate financial markets.
One thing we’re fond of saying is that data science and machine learning aren’t magic. They’re only as good as the data you use, and Tiingo has the best data around, covering everything from forex markets and crypto to financial news. Don’t spend years tinkering with models only to feed them bad information. Go with a source you can trust — go with Tiingo.
Leave a Reply